# Basic Routing
Route::get('/page', function() {
return view('page');
// get is the request method, it may be get, post, put, patch, delete
// view is helper function
// page is the blade template file i.e page.blade.php
});
In above example, view
helper function retrieves a view instance i.e page
is the view blade template file.
open a route file i.e route.php inside routes
directory and paste the above code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Laravel Basic Routing</title>
</head>
<body>
<div>
<h1><center>Laravel Basic Routing</center></h1>
</div>
</body>
</html>
create a file page.blade.php
save it to your resources/views/
directory:
To try basic routing, visit your site using a URL similar to this one:
http://127.0.0.1:8000/page
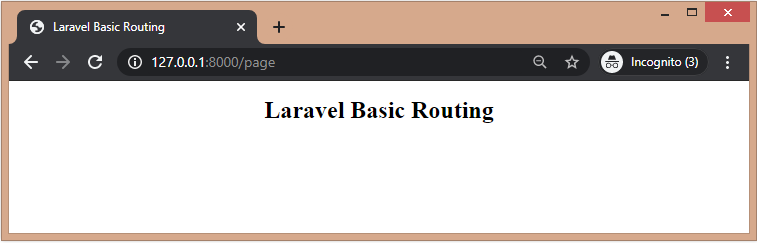
Route::get('product/{id}', function($id) {
echo 'Product Id: '. $id;
});
Route::get('product/{id?}', function($id = 0) {
echo 'Product Id: '. $id;
});
# Regular Expression
Route::get('user/{name}', function($name) {
// executed if {name} is alphabetic characters ;
})->where('name', ['A-Za-z]+');
Route::get('user/{id}', function($id) {
// executed if {id} is numeric
})->where('id', ['0-9]+');
Route::get('user/{id}/{name}', function($id, $name) {
// executed if {id} is numeric and {name} is alphabetic characters
})->where(['id' => '[0-9]+', 'name' => '[a-z]+']);
# Global Constraints
If we want the id
parameter in all route should be integer, so we need to define it as a global constraint. we'll use pattern
method to define global constraint under app/Providers/RouteServiceProvider.php
public function boot()
{
Route::pattern('id', '[0-9]+');
parent::boot();
}
Once the pattern has been defined, it is automatically applied to all routes.
# Redirect Routes
If we want to redirect a url to another url, we can use Route::redirect('/from', '/to');
we want to redirect a url to another url withs status code, we can pass status code as a third parameter. we can use Route::redirect('/from', '/to', 301);
where 301 is status code and 301 is used for permanent redirection.
For permanent redirection, We can use Route::permanentRedirect('/from', '/to');
as well
# Named Routes
We can specify a name for a route by chaining the name
method onto the route definition:
Route::get('user/profile', 'UserController@showProfile')->name('profile');
# Accessing The Current Route
To understand the accessing the current route, we'll use the routing of named route
$route = Route::current();
$route = Route::currentRouteName();
$route = Route::currentRouteAction();