One of the most important feature in laravel is middleware
and it provides the method to filter HTTP
requests. It acts as a bridge between a request
and a response
. In this section, we'll see the middleware's mechanism.
Laravel provides a by default Authenticate
middleware i.e alias auth
under appHttpMiddleware
directory. if we use this middleware, it'll check the user of the application is authenticated or not. If the user is authenticated, it redirects to the home page otherwise redirects to the signin page.
Middleware can be created by executing the following artisan command -
php artisan make:middleware middleware_name
We'll create middleware i.e VoteMiddleware using artisan command.
php artisan make:middleware VoteMiddleware
it will be create under app/Http/Middleware
directory.
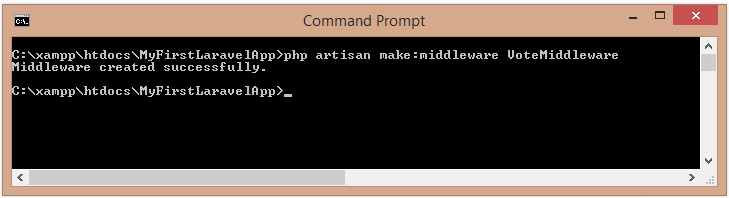
<?php
namespace AppHttpMiddleware;
use Closure;
class VoteMiddleware
{
public function handle($request, Closure $next)
{
return $next($request);
}
}
Laravel provides two types of Middlewares.
- Global Middleware
- Route Middleware
The Global Middleware
are run during every request to your application., where Route Middleware
will be declared to a specific route. The middleware registered at app/Http/Kernel.php
. $middleware property used to register Global Middleware
and $routeMiddleware property is used to register route
specific middleware.
protected $middleware = [
AppHttpMiddlewareTrustProxies::class,
AppHttpMiddlewareCheckForMaintenanceMode::class,
AppHttpMiddlewareValidatePostSize::class,
AppHttpMiddlewareTrimStrings::class,
AppHttpMiddlewareConvertEmptyStringsToNull::class,
];
protected $routeMiddleware = [
'auth' => AppHttpMiddlewareAuthenticate::class,
'auth.basic' => IlluminateAuthMiddlewareAuthenticateWithBasicAuth::class
'bindings' => IlluminateRoutingMiddlewareSubstituteBindings::class,
'cache.headers' => IlluminateHttpMiddlewareSetCacheHeaders::class,
'can' => IlluminateAuthMiddlewareAuthorize::class,
'guest' => AppHttpMiddlewareRedirectIfAuthenticated::class,
'password.confirm' => IlluminateAuthMiddlewareRequirePassword::class,
'signed' => IlluminateRoutingMiddlewareValidateSignature::class,
'throttle' => IlluminateRoutingMiddlewareThrottleRequests::class,
'verified' => IlluminateAuthMiddlewareEnsureEmailIsVerified::class
];
# Example
We've created a VoteMiddleware. now we can now register it in route specific middleware property. Below the code for register the route
Open app/Http/Kernel.php
file and paste the below code
protected $routeMiddleware = [
'auth' => AppHttpMiddlewareAuthenticate::class,
'auth.basic' => IlluminateAuthMiddlewareAuthenticateWithBasicAuth::class
'bindings' => IlluminateRoutingMiddlewareSubstituteBindings::class,
'cache.headers' => IlluminateHttpMiddlewareSetCacheHeaders::class,
'can' => IlluminateAuthMiddlewareAuthorize::class,
'guest' => AppHttpMiddlewareRedirectIfAuthenticated::class,
'password.confirm' => IlluminateAuthMiddlewareRequirePassword::class,
'signed' => IlluminateRoutingMiddlewareValidateSignature::class,
'throttle' => IlluminateRoutingMiddlewareThrottleRequests::class,
'verified' => IlluminateAuthMiddlewareEnsureEmailIsVerified::class,
'Vote' => AppHttpMiddlewareVoteMiddleware::class // register middleware
];
Route::get('vote/{age}', 'UserController@elligible')->middleware('vote');
Route::get('/not-elligible', 'UserController@not_elligible')->name('not_elligible');
In above, we've created the routes to understand the mechanism of middleware.
public function handle($request, Closure $next)
{
$age = $request->segment(2);
if ($age >= 18)
return $next($request);
else
return redirect()->route('not_elligible');
}
Copy the above handle
method and replace it with app/Http/Middleware/VoteMiddleware
's handle method.
To check middleware, visit your site using a URL similar to this one:
http://127.0.0.1:8000/vote/18

visit your site using a URL similar to this one:
http://127.0.0.1:8000/vote/15 i.e second segment i.e 15 should be less than 18
When we navigate to this link, it'll be redirected to not_elligible
named route.
