PHP Flow-Control Statements
In PHP, Control Statements are used for controlling the flow of execution of a program.
Conditional statements, such as if/else and switch, allow a program to execute different pieces of code and loops, such as while, do-while and for, support the repeated execution of code.
Below the several statements in PHP
- if
- if...else
- if...elseif....else
- switch...case
if Statement
if statement is used to execute a block of code only if condition is true
<?php
$num = 15;
if ($num == 15)
echo "Number is equal to 15"; // Output Number is equal to 15
?>
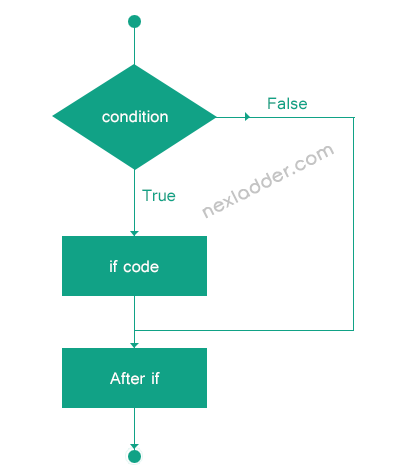
if...else Statement
if...else statement is used to execute a block of code whether condition is true or false
<?php
$num = 15;
if ($num > 10)
echo "Number is greater than 10";
else
echo "Number is less than 10";
// Output Number is greater than 10
?>
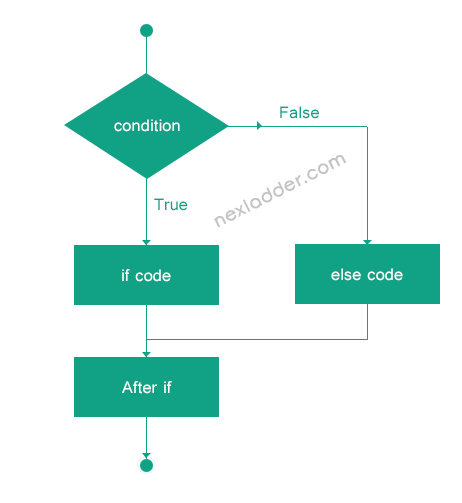
if...elseif...else Statement
if...elseif...else statement is used to executes different codes for more than two conditions.
php
if ($var1 > $var2)
echo "var1 is bigger than var2";
elseif ($var1 == $var2)
echo "var1 is equal to var2";
else
echo "var1 is smaller than var2";
?>
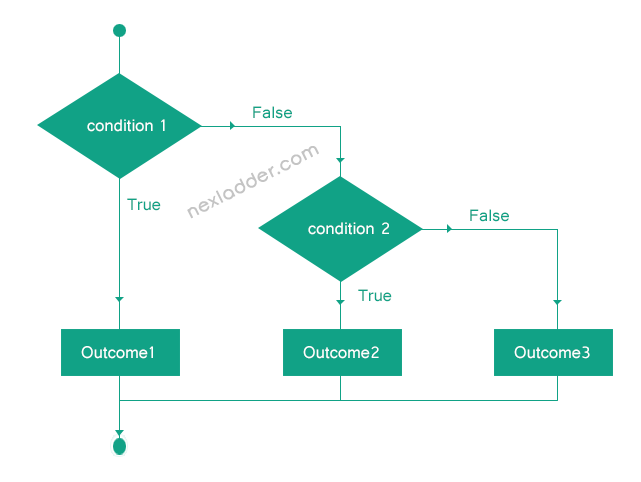